Full Stack Development
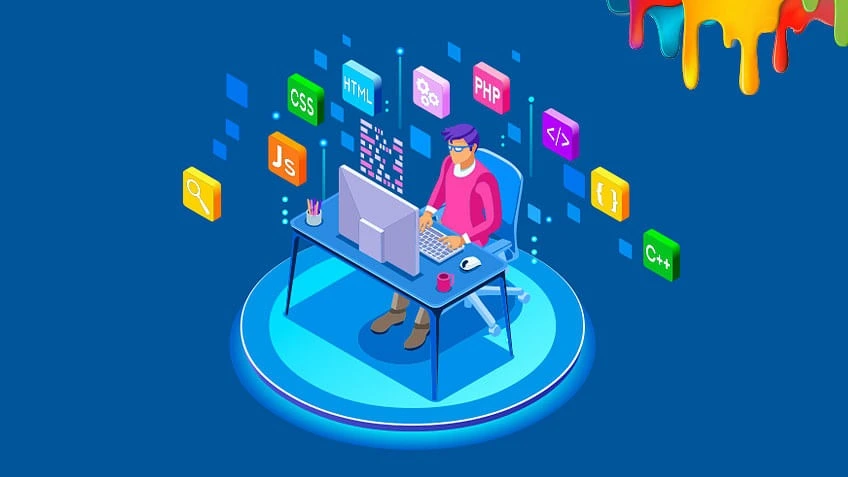
A Full Stack Developer course equips students with a comprehensive grasp of front-end and back-end web development. It covers essential front-end technologies like HTML, CSS, and JavaScript, including frameworks such as React, Angular, or Vue.js for creating dynamic and responsive user interfaces. On the back-end, students learn languages like Node.js, Python, or Java, paired with frameworks like Express, Django, or Spring Boot to build server-side logic, APIs, and manage databases such as MySQL, MongoDB, or PostgreSQL. The curriculum typically includes Git for version control, cloud deployment on platforms like AWS or Azure, and containerization using Docker. Through practical projects, students gain hands-on experience, preparing them to develop and deploy full-scale web applications proficiently as Full Stack Developers.
Duration - 6 Months
Overview of Full Stack Development
Definition and Scope
Roles and Responsibilities of a Full Stack Developer
Front-end vs. Back-end vs. Full Stack Development
Setting Up the Environment
Text Editors and IDEs (VS Code, Sublime Text, WebStorm)
Version Control with Git and GitHub
Introduction to Command Line Interface (CLI)
HTML Basics
Basic Tags and Elements
HTML Document Structure
Semantic HTML
CSS Basics
CSS Syntax and Selectors
Box Model
Styling Text and Fonts
Advanced HTML and CSS
Forms and Validations
CSS Flexbox and Grid Layout
Responsive Web Design and Media Queries
JavaScript Basics
Syntax and Data Types
Operators and Expressions
Conditional Statements and Loops
Advanced JavaScript
Functions and Scope
Asynchronous JavaScript (Callbacks, Promises, async/await)
Error Handling
DOM Manipulation
Selecting and Modifying DOM Elements
Event Handling
Using JavaScript Libraries (e.g., jQuery)
React.js
Introduction to React
Components, Props, and State
React Router for Single Page Applications (SPA)
Managing State with Redux
Alternative Front-end Frameworks (optional)
Vue.js Basics
Angular Basics
Git Basics
Initializing Repositories
Cloning Repositories
Committing Changes
GitHub Collaboration
Branching and Merging
Pull Requests
Issues and Project Management
Introduction to Node.js
What is Node.js?
Setting Up a Node.js Environment
Node.js Modules and npm
Express.js Framework
Setting Up an Express Server
Routing and Middleware
Handling Requests and Responses
Relational Databases (SQL)
Introduction to SQL
CRUD Operations
Using PostgreSQL/MySQL
NoSQL Databases
Introduction to NoSQL and MongoDB
CRUD Operations with MongoDB
Mongoose for MongoDB
Building RESTful APIs
REST Principles
Creating Routes and Controllers
Handling HTTP Methods (GET, POST, PUT, DELETE)
API Authentication and Authorization
JSON Web Tokens (JWT)
OAuth2 Basics
Securing API Endpoints
Connecting Front-end to Back-end
Making HTTP Requests with Fetch and Axios
Handling CORS (Cross-Origin Resource Sharing)
Full Stack Application Development
Building a Complete CRUD Application
Deploying a Full Stack Application
Introduction to Deployment
Deployment Platforms (Heroku, Netlify, Vercel)
Setting Up a CI/CD Pipeline
Server Management and Monitoring
Basics of Linux and Shell Scripting
Using Docker for Containerization
Monitoring Applications with PM2 and Logging
Styling with CSS
Inline, Internal, and External CSS
Basic CSS Properties (color, font, layout, etc.)
Adding Interactivity with JavaScript
Linking JavaScript Files
Basic JavaScript Interactions (onClick, onHover)
Using JavaScript Libraries (jQuery)
Responsive Design Principles
Importance of Responsive Design
Mobile-First Approach
Media Queries
Writing Media Queries
Breakpoints for Different Devices
Flexbox and Grid Layout
Basic Concepts of Flexbox
Basic Concepts of Grid Layout
Practice Exercises for Each Module
Real-world HTML Coding Tasks
Real-world FullStack Tasks
Planning and Designing a Full Stack Application
Implementing the Project
Testing and Deployment
Portfolio Building
Showcasing Projects on GitHub
Creating a Personal Portfolio Website
Preparing for Job Interviews
e