Java
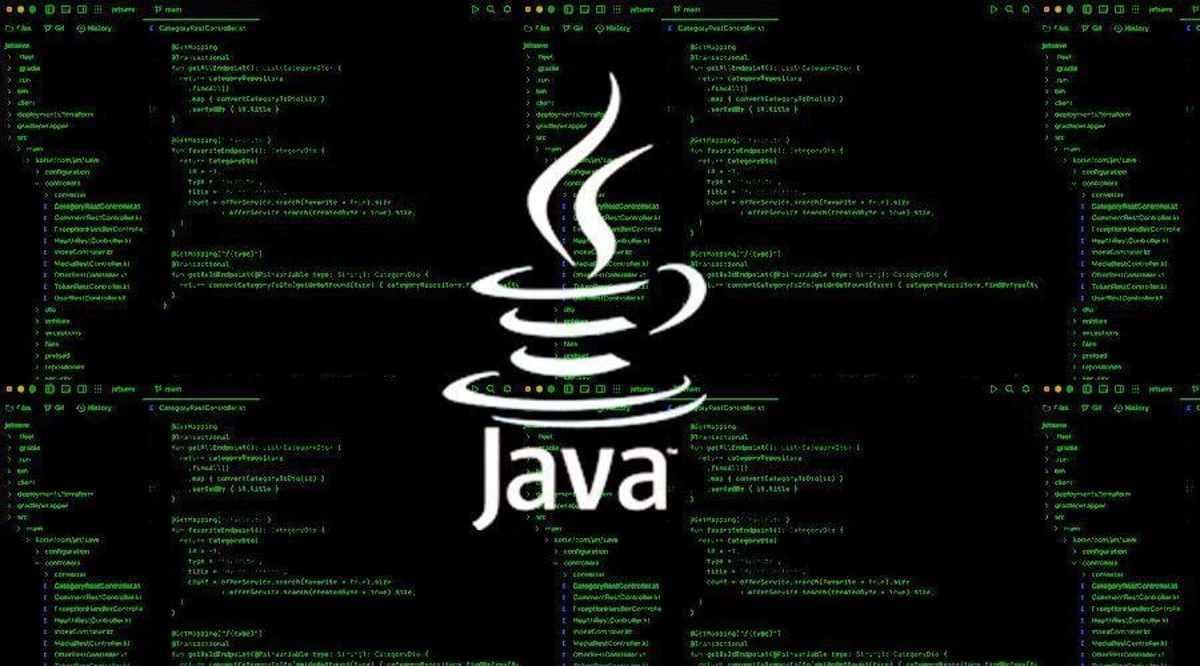
The Java programming course offers an in-depth introduction to Java, focusing on both fundamental and advanced concepts. Starting with the basics of Java syntax, data types, and control structures, the course progresses to object-oriented programming, covering classes, objects, inheritance, polymorphism, and interfaces. Students will learn about exception handling, file I/O, and multithreading, as well as the Java Standard Library. The course includes practical coding exercises, projects, and assessments to reinforce learning and develop problem-solving skills. By the end of the course, students will be proficient in Java, capable of building robust applications, and ready to tackle advanced programming challenges.
Duration - 2 Months
History and Evolution of Java
Features of Java
Java Development Kit (JDK) and Java Runtime Environment (JRE)
Setting Up the Development Environment
Writing, Compiling, and Running a Simple Java Program
Understanding the main() Method
Java Syntax and Structure
Primitive Data Types
Variables and Constants
Type Casting and Type Conversion
Arithmetic Operators
Relational Operators
Logical Operators
Assignment Operators
Unary and Bitwise Operators
If-Else Statements
Switch Case Statements
For Loop
While Loop
Do-While Loop
Enhanced For Loop (for-each)
Nested Loops
Defining and Calling Functions
Function Parameters and Return Values
Scope and Lifetime of Variables
Function Overloading
Inline Functions
Recursive Functions
Single-Dimensional Arrays
Multi-Dimensional Arrays
Array Operations
String Class and String Operations
StringBuffer and StringBuilder Classes
Classes and Objects
Fields and Methods
Constructors
Static Keyword
Inheritance
Polymorphism
Method Overloading and Overriding
Abstract Classes and Interfaces
Defining Packages
Importing Packages
Built-in Packages (java.lang, java.util, etc.)
s
Public, Private, Protected, and Default
Encapsulation and Access Control
Types of Exceptions
Try, Catch, and Finally Blocks
Throw and Throws Keywords
Creating User-defined Exceptions
Handling Multiple Exceptions
File Class and its Methods
Reading from and Writing to Files
BufferedReader and BufferedWriter Classes
Understanding Serialization
Serializable Interface
Deserialization
Overview of the Collections Framework
List, Set, and Map Interfaces
ArrayList, LinkedList
HashSet, TreeSet
HashMap, TreeMap
Iterators and Iteration over Collections
Creating and Running Threads
Thread Lifecycle
Runnable Interface
Synchronization
Inter-thread Communication
Thread Pooling