Python
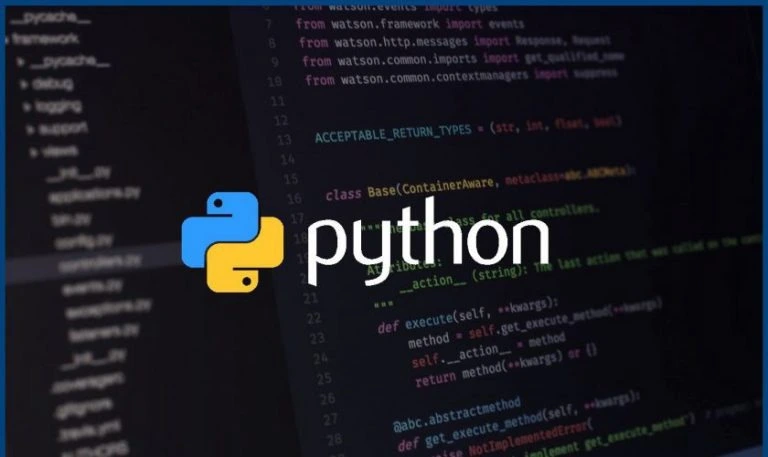
Python is a versatile and widely-used programming language known for its readability and simplicity, making it ideal for beginners and professionals. A comprehensive Python course typically covers fundamental concepts like variables, data types, and control structures such as loops and conditionals. Students then progress to advanced topics like functions, modules, and libraries essential for efficient coding practices. Object-oriented programming (OOP) is a key component, teaching how to create and manage objects and classes. The course includes practical applications in web development, data analysis, machine learning, and automation using tools like Flask, Pandas, NumPy, and TensorFlow. Hands-on projects reinforce learning, equipping students with skills for diverse programming environments.
Duration - 2 Months
History and Evolution
Features and Benefits
Python Applications
Installing Python (Windows, Mac, Linux)
Setting Up an Integrated Development Environment (IDE) (PyCharm, VSCode, Jupyter Notebook)
Writing and Running Your First Python Script
Python Syntax Rules
Writing Comments
Code Indentation
Declaring Variables
Understanding Data Types (int, float, str, bool)
Type Conversion and Casting
Arithmetic Operators
Comparison Operators
Logical Operators
Assignment Operators
Bitwise Operators
Creating Expressions
Operator Precedence and Associativity
If Statements
If-Else Statements
Nested If Statements
For Loop
While Loop
Nested Loops
Break, Continue, and Pass Statements
Creating Lists
Accessing and Modifying List Elements
List Methods (append, remove, sort, etc.)
Creating Tuples
Accessing Tuple Elements
Tuple Operations
Creating Dictionaries
Accessing and Modifying Dictionary Elements
Dictionary Methods (keys, values, items, etc.)
Creating Sets
Set Operations
Set Methods (add, remove, union, intersection, etc.)
Function Syntax
Calling Functions
Function Arguments and Return Values
Default and Keyword Arguments
Lambda Functions
Scope and Lifetime of Variables
Importing Modules
Standard Library Modules (math, datetime, random, etc.)
Creating and Using Custom Modules
Introduction to Packages
Creating and Using Packages
Opening and Closing Files
Reading from Files
Writing to Files
Working with File Paths
read(), readline(), readlines()
write(), writelines()
seek(), tell()
Understanding Exceptions
Try and Except Blocks
Finally Block
Handling Multiple Exceptions
Raising Exceptions
Creating Custom Exceptions
Understanding Classes and Objects
Creating and Using Classes
Instance Variables and Methods
Constructors (init method)
Inheritance
Method Overriding
Encapsulation
Introduction to NumPy
Introduction to Pandas
Introduction to Matplotlib
Installing Libraries with pip
Importing and Using Libraries
Plotting with Matplotlib
Creating Basic Charts (Line, Bar, Histogram)
Customizing Plots (Titles, Labels, Legends)
Understanding Python Scripts
Writing Simple Automation Scripts
Scheduling Scripts
Practice Exercises for Each Module
Real-world Problem Solving
Building Small Python Applications
Implementing Data Processing Pipelines